Overview
Learn how you can build any financial use case in Southeast Asia with our APIs.
Overview
Overview
Learn how you can build any financial use case in Southeast Asia with our APIs.

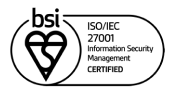

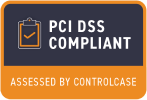
Brankas’ products are ISO 270001 and PCI-DSS (Payment Card Industry Data Security Standard) certified to ensure the highest security standards for customers.
We also maintain a Privacy Policy to transparently provide you information about how your data is used.
See Brankas Privacy PolicyGetting Started
Getting Started
Guides and examples to get you started and integrated with Brankas.
Products
Products
Guides and examples to get you started and integrated with Brankas.
API Aggregation
Business Operations
Payments
Financial Infrastructure
Open Core
Build a reliable financial ecosystem with a scalable and secure banking solution.
Open Finance Suite
Receive money with a powerful, custom-made and secure payment infrastructure.
Merchant Link
Create new revenue streams and accelerate your business with world-class technology.
Money-in Yokke!
Accept payments and scale faster
Tap
Tap
Learn how you can speed up your integration with Brankas Tap.
Updated 7 months ago